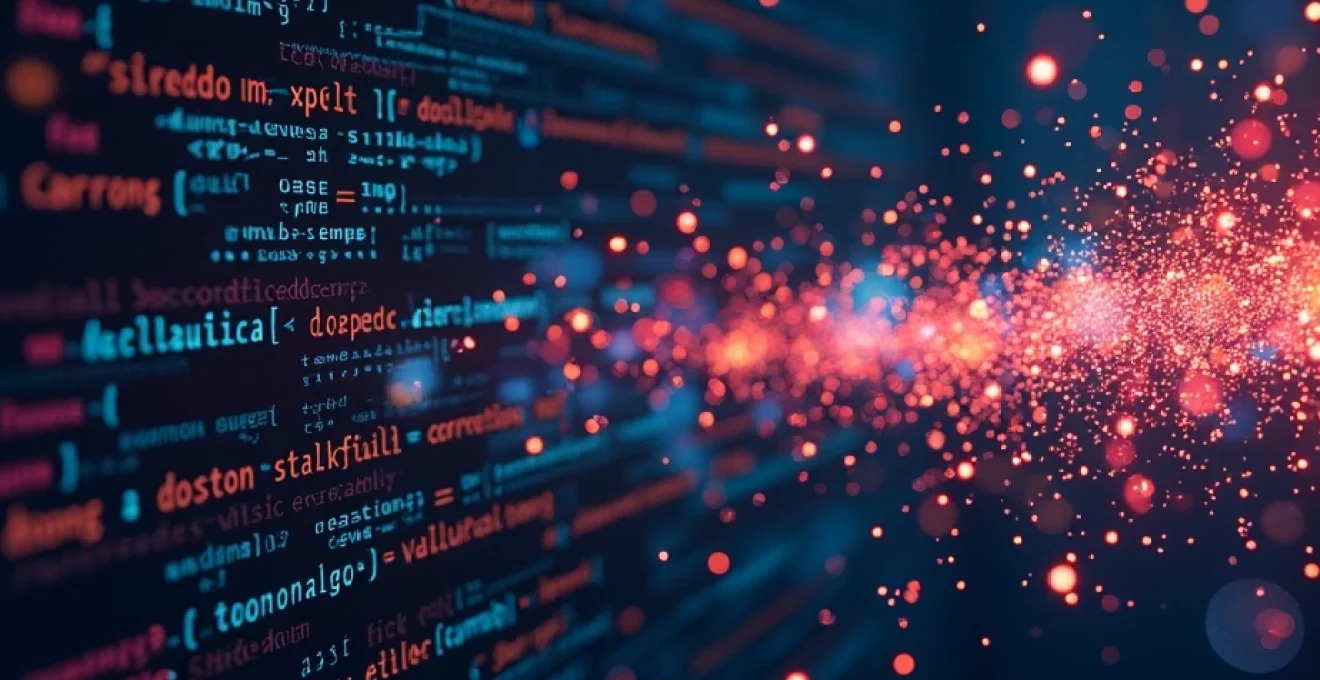
Source code forms the foundation of all software applications, serving as the blueprint that dictates how a program functions. It's the human-readable set of instructions that programmers write to create everything from simple mobile apps to complex operating systems. As technology continues to evolve, understanding source code becomes increasingly crucial for developers, engineers, and even business leaders who want to grasp the intricacies of their digital products.
In this comprehensive exploration of source code, we'll delve into its fundamental concepts, architecture, management practices, and the critical role it plays in modern software development. You'll gain insights into how source code is structured, maintained, and optimized to create robust, efficient, and secure software solutions that drive innovation across industries.
Fundamentals of source code in programming languages
At its core, source code is a set of instructions written in a programming language that humans can read and understand. These instructions are then translated into machine code that computers can execute. Different programming languages offer various approaches to writing source code, each with its own syntax, semantics, and paradigms.
High-level programming languages like Python, Java, and C++ allow developers to write code that's closer to human language, making it easier to read and write. On the other hand, low-level languages like Assembly provide more direct control over hardware but are more challenging to work with. The choice of programming language often depends on the specific requirements of the project, such as performance needs, target platform, and developer expertise.
One of the key aspects of source code is its readability. Well-written code should be clear and understandable not only to the original author but also to other developers who may need to maintain or modify it in the future. This is where coding standards and best practices come into play, ensuring consistency and maintainability across large codebases.
Source code architecture and organization
The architecture and organization of source code play a crucial role in determining the scalability, maintainability, and overall quality of a software project. As applications grow in complexity, it becomes increasingly important to structure code in a way that promotes modularity, reusability, and ease of understanding.
Modular design patterns in object-oriented programming
Object-Oriented Programming (OOP) is a paradigm that organizes code into reusable, self-contained objects. This approach allows developers to create modular designs that encapsulate data and behavior, making it easier to manage complex systems. Design patterns, such as the Model-View-Controller (MVC) pattern, provide proven solutions to common architectural challenges in OOP.
By utilizing these patterns, you can create more flexible and maintainable code structures. For example, the Factory pattern allows for the creation of objects without specifying their exact class, promoting loose coupling between components. Similarly, the Observer pattern enables a one-to-many dependency between objects, facilitating event-driven programming.
Functional programming paradigms and code structure
Functional programming is another paradigm that has gained popularity in recent years, especially for handling complex data transformations and concurrent operations. Languages like Haskell and Scala, as well as functional features in languages like JavaScript and Python, encourage a style of programming that treats computation as the evaluation of mathematical functions.
In functional programming, code is organized around pure functions that avoid changing state and mutable data. This approach can lead to more predictable and testable code, as functions always produce the same output for a given input. Concepts like immutability and higher-order functions are central to functional programming, influencing how source code is structured and organized.
Microservices architecture in distributed systems
Microservices architecture has emerged as a powerful approach for building large-scale, distributed systems. This architectural style structures an application as a collection of loosely coupled services, each running in its own process and communicating through lightweight mechanisms, often HTTP-based APIs.
When implementing microservices, source code is typically organized into separate repositories or modules for each service. This separation allows teams to work independently on different services, choose the most appropriate technology stack for each service, and deploy updates more frequently. However, it also introduces challenges in terms of data consistency and inter-service communication that need to be carefully addressed in the source code.
Domain-driven design (DDD) for complex software projects
Domain-Driven Design is an approach to software development that focuses on creating a shared understanding of the problem domain among developers and domain experts. In DDD, the structure and language of the source code closely reflect the business domain, making the code more intuitive and aligned with business needs.
Key concepts in DDD, such as bounded contexts and aggregates, influence how source code is organized. For instance, you might structure your code around domain entities and value objects, with clear boundaries between different subdomains. This approach can lead to more maintainable and adaptable software systems, especially for complex business domains.
Version control systems for source code management
Version control systems (VCS) are essential tools for managing source code in modern software development. They allow developers to track changes, collaborate effectively, and maintain a history of code modifications. Understanding how to use VCS effectively is crucial for any software development team.
Git workflows and branching strategies
Git has become the de facto standard for version control in software development. Its distributed nature and powerful branching capabilities make it ideal for managing complex projects with multiple contributors. Effective use of Git relies on adopting a suitable workflow and branching strategy.
The Gitflow workflow, for example, defines a strict branching model designed around project releases. It involves maintaining separate branches for features, releases, and hotfixes, with a main branch representing the official release history. This approach helps teams manage concurrent development and maintain a clean project history.
Distributed version control with mercurial
While Git dominates the version control landscape, Mercurial is another distributed VCS that offers similar functionality with a focus on simplicity and ease of use. Mercurial's approach to branching and merging can be more intuitive for some developers, making it a viable alternative for certain projects.
Mercurial's named branches provide a straightforward way to manage parallel lines of development. This feature, combined with Mercurial's emphasis on immutable history, can lead to clearer project timelines and easier collaboration among team members.
Subversion (SVN) for centralized source control
Although distributed version control systems have largely superseded centralized systems, Subversion (SVN) still finds use in some development environments. SVN's centralized model can be simpler to understand and manage for smaller teams or projects with linear development processes.
One advantage of SVN is its handling of large binary files and projects with extensive history. Its centralized nature means that developers don't need to clone the entire repository history, which can be beneficial for projects with large assets or long-running histories.
Code review processes using GitHub pull requests
Code reviews are a critical part of maintaining code quality and fostering knowledge sharing within development teams. GitHub's pull request feature has become a standard tool for facilitating code reviews in many organizations.
Pull requests allow developers to propose changes to a codebase, discuss those changes with teammates, and merge the changes once approved. This process not only improves code quality but also serves as a learning opportunity for team members. Effective use of pull requests involves clear communication, thorough reviews, and timely feedback to maintain development velocity.
Compilation and interpretation of source code
The process of turning source code into executable programs involves either compilation or interpretation, depending on the programming language and environment. Understanding these processes is crucial for optimizing performance and debugging issues in software applications.
Compiled languages like C++ and Rust translate source code directly into machine code that can be executed by the computer's processor. This compilation process typically results in faster execution times but requires a separate build step before the program can be run. Interpreted languages like Python and JavaScript, on the other hand, execute code line by line at runtime, offering more flexibility but potentially slower performance for certain tasks.
Many modern languages use a hybrid approach, combining compilation and interpretation. For example, Java compiles source code into bytecode, which is then interpreted by the Java Virtual Machine (JVM). This approach offers a balance between performance and platform independence.
Source code analysis and quality assurance
Ensuring the quality and reliability of source code is paramount in software development. Various tools and techniques are employed to analyze code, identify potential issues, and maintain high standards of quality.
Static code analysis tools: SonarQube and ESLint
Static code analysis tools examine source code without executing it, identifying potential bugs, security vulnerabilities, and code smells. SonarQube is a popular platform that provides continuous inspection of code quality, offering insights into areas such as code duplication, complexity, and test coverage.
For JavaScript development, ESLint has become an indispensable tool for enforcing coding standards and catching potential errors early in the development process. Its highly configurable nature allows teams to define and enforce custom rules that align with their specific coding practices and standards.
Dynamic analysis techniques for runtime behavior
While static analysis is valuable, dynamic analysis techniques provide insights into how code behaves during execution. Profiling tools can help identify performance bottlenecks, memory leaks, and other runtime issues that may not be apparent from static analysis alone.
Techniques such as code instrumentation and runtime monitoring allow developers to gather detailed information about program behavior, resource usage, and potential security vulnerabilities as the application runs. This data is invaluable for optimizing performance and ensuring the reliability of complex systems.
Cyclomatic complexity and code maintainability metrics
Cyclomatic complexity is a metric that measures the number of linearly independent paths through a program's source code. High cyclomatic complexity often indicates code that is difficult to understand, test, and maintain. By monitoring this metric, development teams can identify areas of code that may need refactoring or simplification.
Other maintainability metrics, such as the maintainability index and cognitive complexity, provide additional insights into code quality. These metrics help teams assess the long-term sustainability of their codebase and make informed decisions about where to focus refactoring efforts.
Test-driven development (TDD) and unit testing frameworks
Test-Driven Development is a software development approach that emphasizes writing tests before implementing functionality. This practice not only ensures comprehensive test coverage but also encourages developers to think critically about code design and functionality from the outset.
Unit testing frameworks like JUnit for Java, pytest for Python, and Jest for JavaScript provide the tools necessary to implement TDD effectively. These frameworks allow developers to write and run automated tests, ensuring that individual components of the codebase function correctly in isolation.
Source code security and vulnerability management
In an era of increasing cyber threats, securing source code and managing vulnerabilities have become critical aspects of software development. Developers must be vigilant in identifying and addressing potential security risks throughout the development lifecycle.
Static Application Security Testing (SAST) tools analyze source code to identify potential security vulnerabilities before the application is deployed. These tools can detect issues such as SQL injection vulnerabilities, cross-site scripting (XSS) risks, and insecure cryptographic practices.
Additionally, dependency management is crucial for maintaining secure codebases. Tools like npm audit for JavaScript projects and OWASP Dependency-Check for Java applications help identify and mitigate vulnerabilities in third-party libraries and components used in the project.
Implementing secure coding practices, such as input validation, proper error handling, and secure authentication mechanisms, is essential for building robust and secure applications. Regular security audits and penetration testing can help identify vulnerabilities that may have been missed during development.
By integrating security considerations into every stage of the development process, from initial design to ongoing maintenance, you can significantly reduce the risk of security breaches and protect sensitive data handled by your applications.